Examples
Detail view examples
Error!
Success!
jsmithhh
User profile data
-
Avatar
-
Namejsmithhh
-
Emailjsmith@example.com
-
Active
-
Typeadmin
-
Created2 years ago
-
Updated2 days ago
<?php
namespace App\Http\Livewire\DetailView;
use App\Models\User;
use LaravelViews\Facades\UI;
use LaravelViews\Views\DetailView;
class UserDetailView extends DetailView
{
protected $modelClass = User::class;
public function heading(User $model)
{
return [$model->name, 'User profile data'];
}
/**
* @param $model Model instance
* @return Array Array with all the detail data or the components
*/
public function detail(User $model)
{
return [
'Avatar' => UI::avatar(asset('storage/' . $model->avatar)),
'Name' => $model->name,
'Email' => $model->email,
'Active' => $model->active ? UI::icon('check', 'success') : UI::icon('slash', 'danger'),
'Type' => $model->type,
'Created' => $model->created_at->diffForHumans(),
'Updated' => $model->updated_at->diffForHumans(),
];
}
}
Error!
Success!
jsmithhh
User profile data
Change user as admin
Change user as writer
Activate / Deactivate
-
Avatar
-
Namejsmithhh
-
Emailjsmith@example.com
-
Active
-
Typeadmin
-
Created2 years ago
-
Updated2 days ago
<?php
namespace App\Http\Livewire\DetailView;
use App\Actions\ChangeUserAsAdmin;
use App\Actions\ChangeUserAsWriter;
use App\Actions\ToggleUserAction;
class UserDetailViewWithActions extends UserDetailView
{
public function actions()
{
return [
new ChangeUserAsAdmin,
new ChangeUserAsWriter,
new ToggleUserAction,
];
}
}
Error!
Success!
jsmithhh
User profile data
Change user as admin
Change user as writer
Activate / Deactivate
Name
jsmithhh
Email
jsmith@example.com
Active
1
Type
Admin
Created
2 years ago
Updated
2 days ago
<?php
namespace App\Http\Livewire\DetailView;
class UserDetailViewWithCustomComponent extends UserDetailViewWithActions
{
protected $detailComponent = 'components.user-detail';
/**
* @param $model Model instance
* @return Array Array with all the detail data or the components
*/
public function detail($model)
{
return [
'user' => $model,
];
}
}
Error!
Success!
jsmithhh
User profile data
Change user as admin
Change user as writer
Activate / Deactivate
230k
Sales
230k
Sales
230k
Sales
-
Avatar
-
Namejsmithhh
-
Emailjsmith@example.com
-
Active
-
TypeAdmin
-
Created2 years ago
-
Updated2 days ago
Gallery
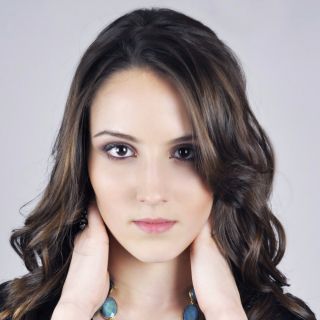
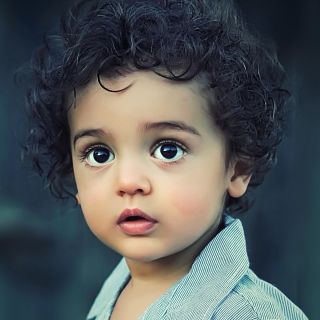
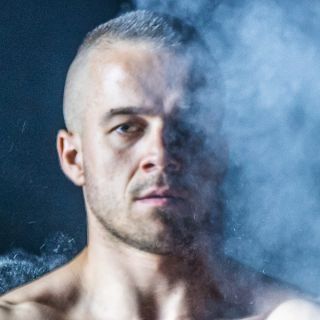
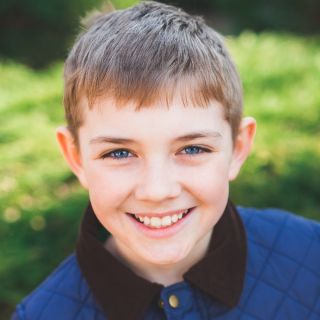
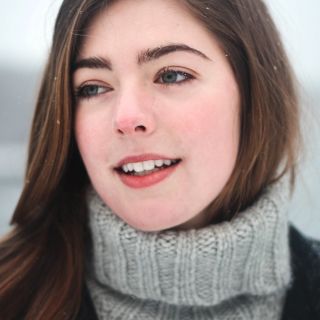
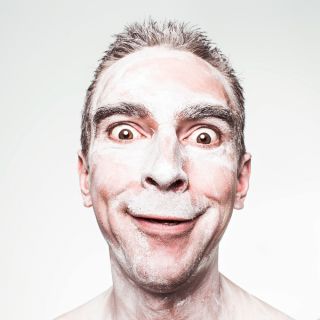
<?php
namespace App\Http\Livewire\DetailView;
use LaravelViews\Facades\UI;
class UserDetailViewWithMultipleComponents extends UserDetailViewWithActions
{
/**
* @param $model Model instance
* @return Array Array with all the detail data or the components
*/
public function detail($model)
{
return [
UI::component('components.metrics', []),
UI::attributes([
'Avatar' => UI::avatar(asset('storage/' . $model->avatar)),
'Name' => $model->name,
'Email' => $model->email,
'Active' => $model->active ? UI::icon('check', 'success') : UI::icon('slash', 'danger'),
'Type' => ucfirst($model->type),
'Created' => $model->created_at->diffForHumans(),
'Updated' => $model->updated_at->diffForHumans(),
]),
UI::component('components.gallery', []),
];
}
}